Advanced Examples
Preface
As advanced examples, we are going to use the following tutorials from LangGraph:
We are also not going to focus on a basic usage - the main focus is going to be on langgraph_compare.jsons_to_csv.GraphConfig
.
If You want to check basic usage, refer to: Getting Started.
Multi-Agent Network
This example is based on Agent Architectures - Multi-Agent Network and expands on Exporting JSON’s to CSV from Getting Started.
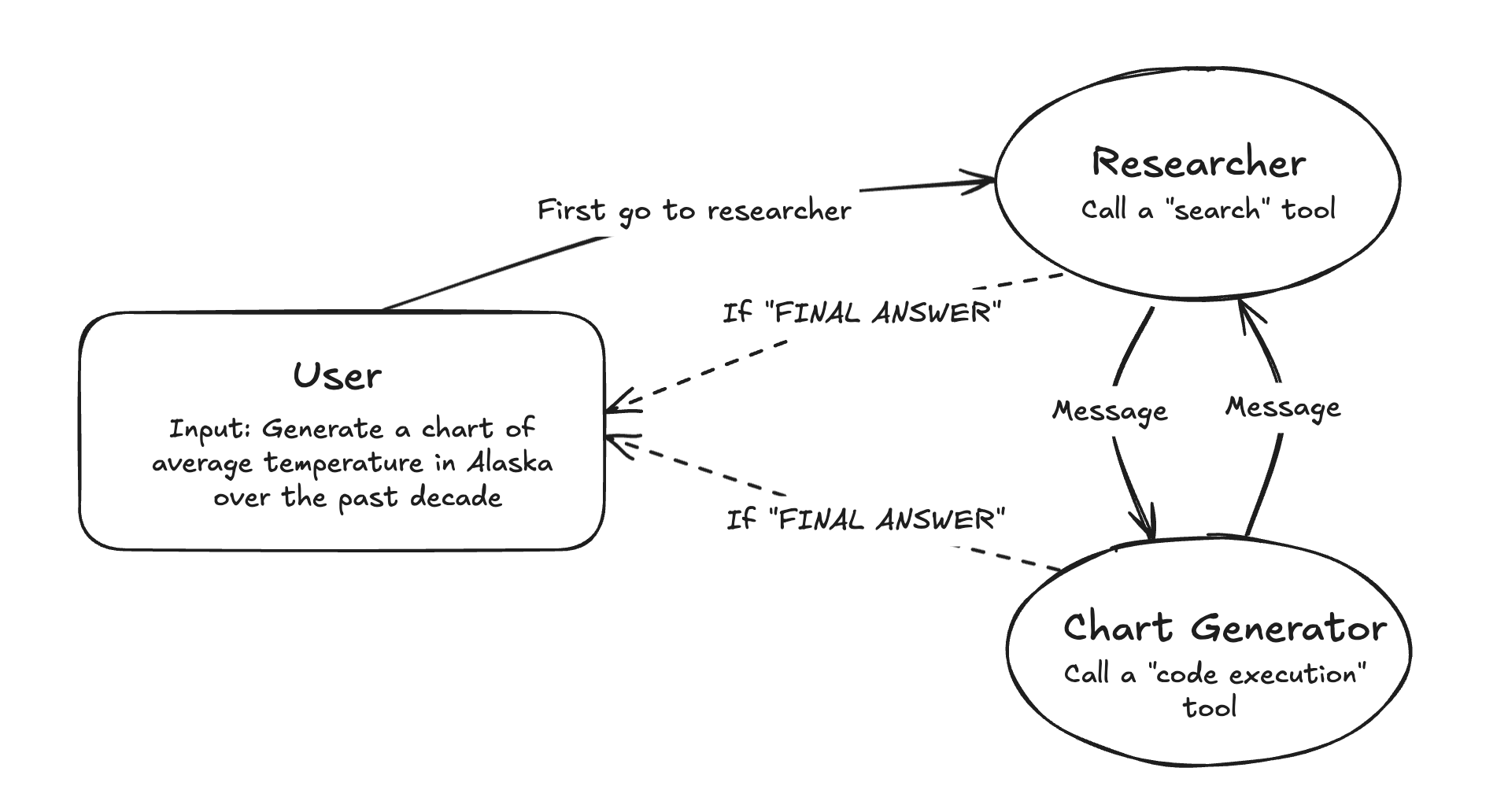
The premise of this example is to show that GraphConfig
can have multiple nodes.
In case of this example - we will have 2 nodes - researcher
, chart_generator
.
Example:
# Needed imports
from langgraph_compare.experiment import create_experiment
from langgraph_compare.jsons_to_csv import GraphConfig, export_jsons_to_csv
# Init for experiment project structure
exp = create_experiment("test")
# We can add multiple nodes!
graph_config = GraphConfig(
nodes=['researcher','chart_generator']
)
# You can provide You own file name as an optional attribute csv_path.
# Otherwise it will use the default file name - "csv_output.csv"
export_jsons_to_csv(exp, graph_config)
Multi-Agent Supervisor
This example is based on Agent Architectures - Multi-Agent Supervisor. It introduces the concept of a Supervisor
- a node that controls other nodes.
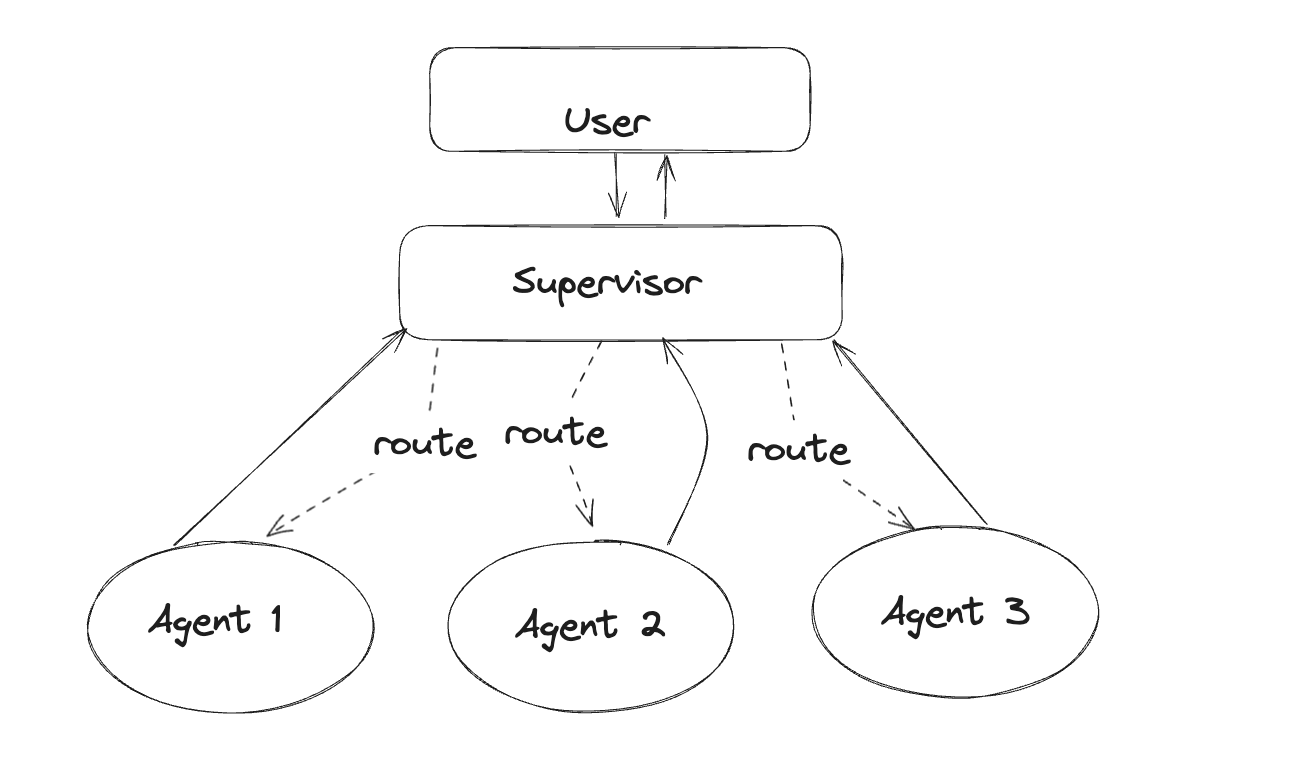
In this example, we will introduce langgraph_compare.jsons_to_csv.SupervisorConfig
. It will supervise the graph - working more or less work the same as GraphConfig
. The concept of supervisors will make more sense in Hierarchical Agent Teams.
Example:
# Needed imports
from langgraph_compare.experiment import create_experiment
from langgraph_compare.jsons_to_csv import GraphConfig, SupervisorConfig, export_jsons_to_csv
# Init for experiment project structure
exp = create_experiment("test")
# Supervisor for graph
supervisor = SupervisorConfig(
name="supervisor",
supervisor_type="graph"
)
# Config with supervisor and additional nodes
graph_config = GraphConfig(
supervisors=[supervisor],
nodes=["Researcher", "Coder"]
)
# You can provide You own file name as an optional attribute csv_path.
# Otherwise it will use the default file name - "csv_output.csv"
export_jsons_to_csv(exp, graph_config)
Hierarchical Agent Teams
This example is based on Agent Architectures - Hierarchical Agent Teams. It introduces the concept of a SubgraphConfig
- a node that controls other nodes.
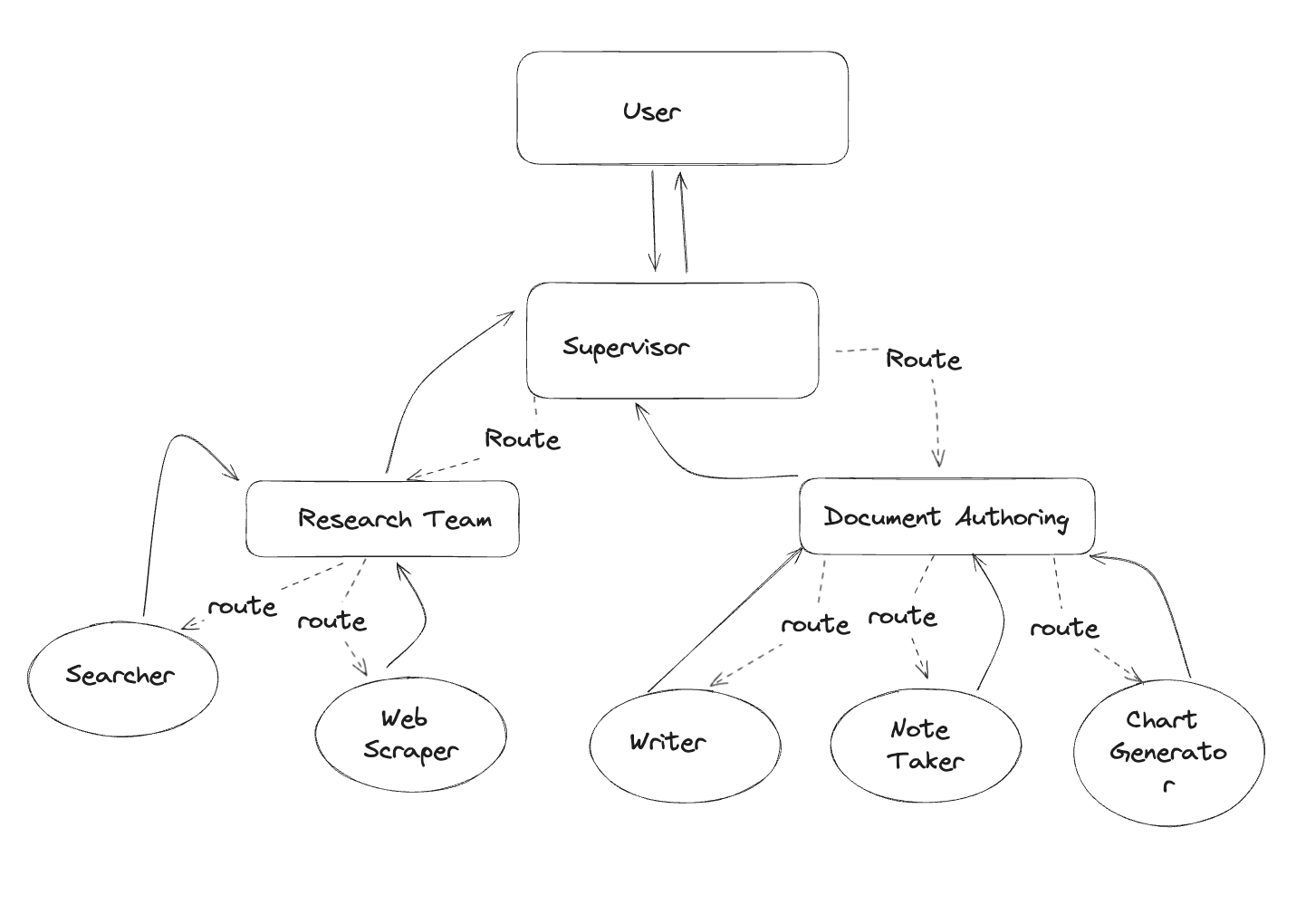
In this example, we have a Graph
that is build from two SubGraphs
. Those graphs are controlled by a Supervisor
- that routes traffic to subgraphs.
Furthermore, every graph has its own supervisor - that controls what is happening inside of it.
IMPORTANT: Be sure to call supervisors with different names - so you can differentiate between them! Calling supervisors with the same names WILL brake the parser.
Example:
# Needed imports
from langgraph_compare.experiment import create_experiment
from langgraph_compare.jsons_to_csv import GraphConfig, SubgraphConfig, SupervisorConfig, export_jsons_to_csv
# Init for experiment project structure
exp = create_experiment("test")
# Config for entire graph supervisor
graph_supervisor = SupervisorConfig(
name="graph_supervisor",
supervisor_type="graph"
)
# Config for Research Team subgraph supervisor
research_supervisor = SupervisorConfig(
name="research_supervisor",
supervisor_type="subgraph"
)
# Config for Paper Writing Team subgraph supervisor
paper_supervisor = SupervisorConfig(
name="paper_supervisor",
supervisor_type="subgraph"
)
# Config for Research Team subgraph
research_team = SubgraphConfig(
name="ResearchTeam",
nodes=["Search", "WebScraper"],
supervisor=research_supervisor
)
# Config for Paper Writing Team subgraph
paper_team = SubgraphConfig(
name="PaperWritingTeam",
nodes=["DocWriter", "NoteTaker","ChartGenerator"],
supervisor=paper_supervisor
)
# Config for complete graph
graph_config = GraphConfig(
supervisors=[graph_supervisor],
subgraphs=[research_team, paper_supervisor]
)
# You can provide You own file name as an optional attribute csv_path.
# Otherwise it will use the default file name - "csv_output.csv"
export_jsons_to_csv(exp, graph_config)
Notice how:
every supervisor has a config (both graph and subgraphs) - but they have a different
supervisor_type
.every graph has a config (both graph and subgraphs) - but they are using different classes:
GraphConfig
orSubgraphConfig
GraphConfig
doesn’t havenodes
defined - since they are being taken care of by subgraphs.